Camera Preview
Showing camera preview in HTML
Requires Cordova plugin: https://github.com/cordova-plugin-camera-preview/cordova-plugin-camera-preview.git
. For more info, please see the Cordova Camera Preview docs.
https://github.com/cordova-plugin-camera-preview/cordova-plugin-camera-preview
Stuck on a Cordova issue?
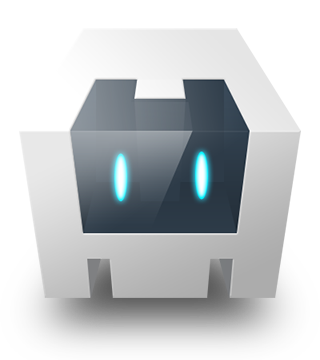
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionic’s experts offer premium advisory services for both community plugins and premier plugins.
Installation
- Capacitor
- Cordova
- Enterprise
$ npm install cordova-plugin-camera-preview
$ npm install @awesome-cordova-plugins/camera-preview
$ ionic cap sync
$ ionic cordova plugin add cordova-plugin-camera-preview
$ npm install @awesome-cordova-plugins/camera-preview
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Learn More or if you're interested in an enterprise version of this plugin Contact Us
Supported Platforms
- Android
- iOS
Usage
React
Learn more about using Ionic Native components in React
Angular
import { CameraPreview, CameraPreviewPictureOptions, CameraPreviewOptions, CameraPreviewDimensions } from '@awesome-cordova-plugins/camera-preview/ngx';
constructor(private cameraPreview: CameraPreview) { }
...
// camera options (Size and location). In the following example, the preview uses the rear camera and display the preview in the back of the webview
const cameraPreviewOpts: CameraPreviewOptions = {
x: 0,
y: 0,
width: window.screen.width,
height: window.screen.height,
camera: 'rear',
tapPhoto: true,
previewDrag: true,
toBack: true,
alpha: 1
}
// start camera
this.cameraPreview.startCamera(cameraPreviewOpts).then(
(res) => {
console.log(res)
},
(err) => {
console.log(err)
});
// Set the handler to run every time we take a picture
this.cameraPreview.setOnPictureTakenHandler().subscribe((result) => {
console.log(result);
// do something with the result
});
// picture options
const pictureOpts: CameraPreviewPictureOptions = {
width: 1280,
height: 1280,
quality: 85
}
// take a picture
this.cameraPreview.takePicture(this.pictureOpts).then((imageData) => {
this.picture = 'data:image/jpeg;base64,' + imageData;
}, (err) => {
console.log(err);
this.picture = 'assets/img/test.jpg';
});
// take a snap shot
this.cameraPreview.takeSnapshot(this.pictureOpts).then((imageData) => {
this.picture = 'data:image/jpeg;base64,' + imageData;
}, (err) => {
console.log(err);
this.picture = 'assets/img/test.jpg';
});
// Switch camera
this.cameraPreview.switchCamera();
// set color effect to negative
this.cameraPreview.setColorEffect('negative');
// Stop the camera preview
this.cameraPreview.stopCamera();