Background Fetch
iOS Background Fetch Implementation. See: https://developer.apple.com/reference/uikit/uiapplication#1657399 iOS Background Fetch is basically an API which wakes up your app about every 15 minutes (during the user's prime-time hours) and provides your app exactly 30s of background running-time. This plugin will execute your provided callbackFn whenever a background-fetch event occurs. There is no way to increase the rate which a fetch-event occurs and this plugin sets the rate to the most frequent possible value of UIApplicationBackgroundFetchIntervalMinimum -- iOS determines the rate automatically based upon device usage and time-of-day (ie: fetch-rate is about ~15min during prime-time hours; less frequently when the user is presumed to be sleeping, at 3am for example). For more detail, please see https://github.com/transistorsoft/cordova-plugin-background-fetch
https://github.com/transistorsoft/cordova-plugin-background-fetch
Stuck on a Cordova issue?
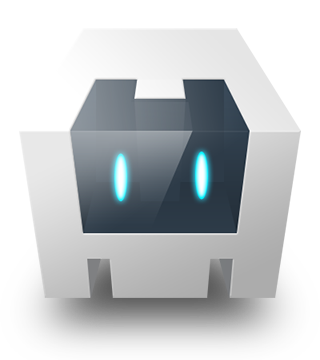
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionic’s experts offer premium advisory services for both community plugins and premier plugins.
Installation
- Capacitor
- Cordova
- Enterprise
$ npm install cordova-plugin-background-fetch
$ npm install @awesome-cordova-plugins/background-fetch
$ ionic cap sync
$ ionic cordova plugin add cordova-plugin-background-fetch
$ npm install @awesome-cordova-plugins/background-fetch
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Learn More or if you're interested in an enterprise version of this plugin Contact Us
Supported Platforms
- iOS
Usage
React
Learn more about using Ionic Native components in React
Angular
import { BackgroundFetch, BackgroundFetchConfig } from '@awesome-cordova-plugins/background-fetch/ngx';
constructor(private backgroundFetch: BackgroundFetch) {
const config: BackgroundFetchConfig = {
stopOnTerminate: false, // Set true to cease background-fetch from operating after user "closes" the app. Defaults to true.
}
backgroundFetch.configure(config)
.then(() => {
console.log('Background Fetch initialized');
this.backgroundFetch.finish();
})
.catch(e => console.log('Error initializing background fetch', e));
// Start the background-fetch API. Your callbackFn provided to #configure will be executed each time a background-fetch event occurs. NOTE the #configure method automatically calls #start. You do not have to call this method after you #configure the plugin
backgroundFetch.start();
// Stop the background-fetch API from firing fetch events. Your callbackFn provided to #configure will no longer be executed.
backgroundFetch.stop();
}